Which frontend library should you choose for your project? You've heard good things of Vue, but on the other hand React is the industry standard. I've recently worked with both frameworks, and in this post I'll compare the two options and share my opinionated and honest thoughts.
1. Performance
Both Vue and React handle rendering with virtual DOMs, but they use different approaches. React uses its Fiber Architecture for efficient updates and handling of asynchronous tasks. Vue has its own reactive system that automatically tracks dependencies and optimizes re-rendering by ensuring only necessary DOM updates occur.
Outcome: Vue may offer slight performance advantages in specific scenarios, but both frameworks provide efficient performance for most web applications, so there's not much difference here.
2. Syntax and Semantics
Vue
Vue offers a structured and intuitive syntax with clear separation of concerns, but comes with its own learning curves:
Advantages:
- Clear template directives like v-if and v-for make logic easy to read
- Separation of <template>, <script>, and <style> in single-file components provides clear organization
Challenges:
Multiple shorthand syntaxes to learn:
- :prop is shorthand for v-bind:prop
- @click is shorthand for v-on:click
- v-model is syntactic sugar for :modelValue and @update:modelValue
- string-like references to variables in templates can be confusing
Single-file components must be defined in separate files, making it hard (without using tools or JSX) to declare multiple reusable components in the same file. This can lead to many small files in your project or alternatively too much copy paste code.
Two different API styles to choose from:
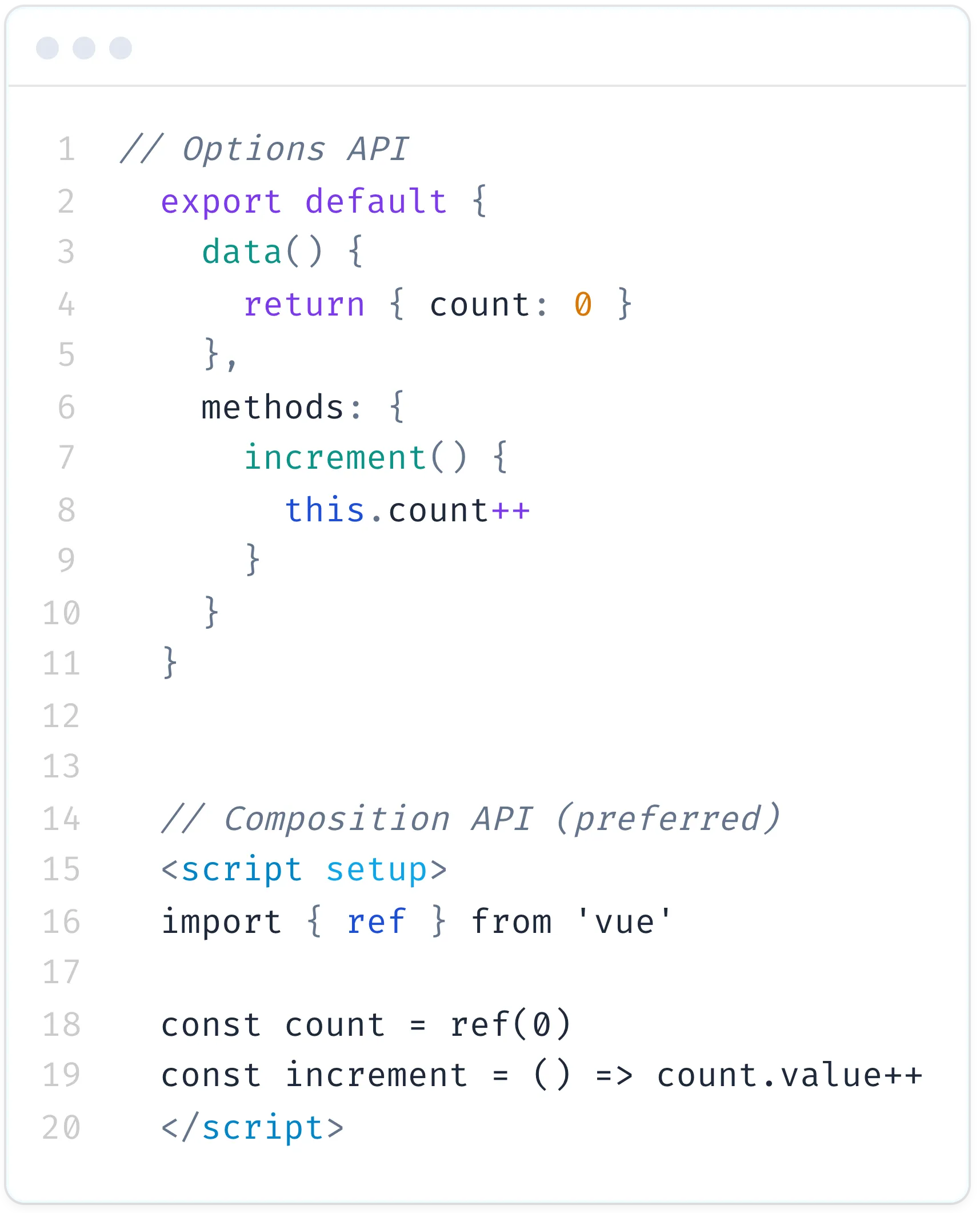
Multiple ways to define props:
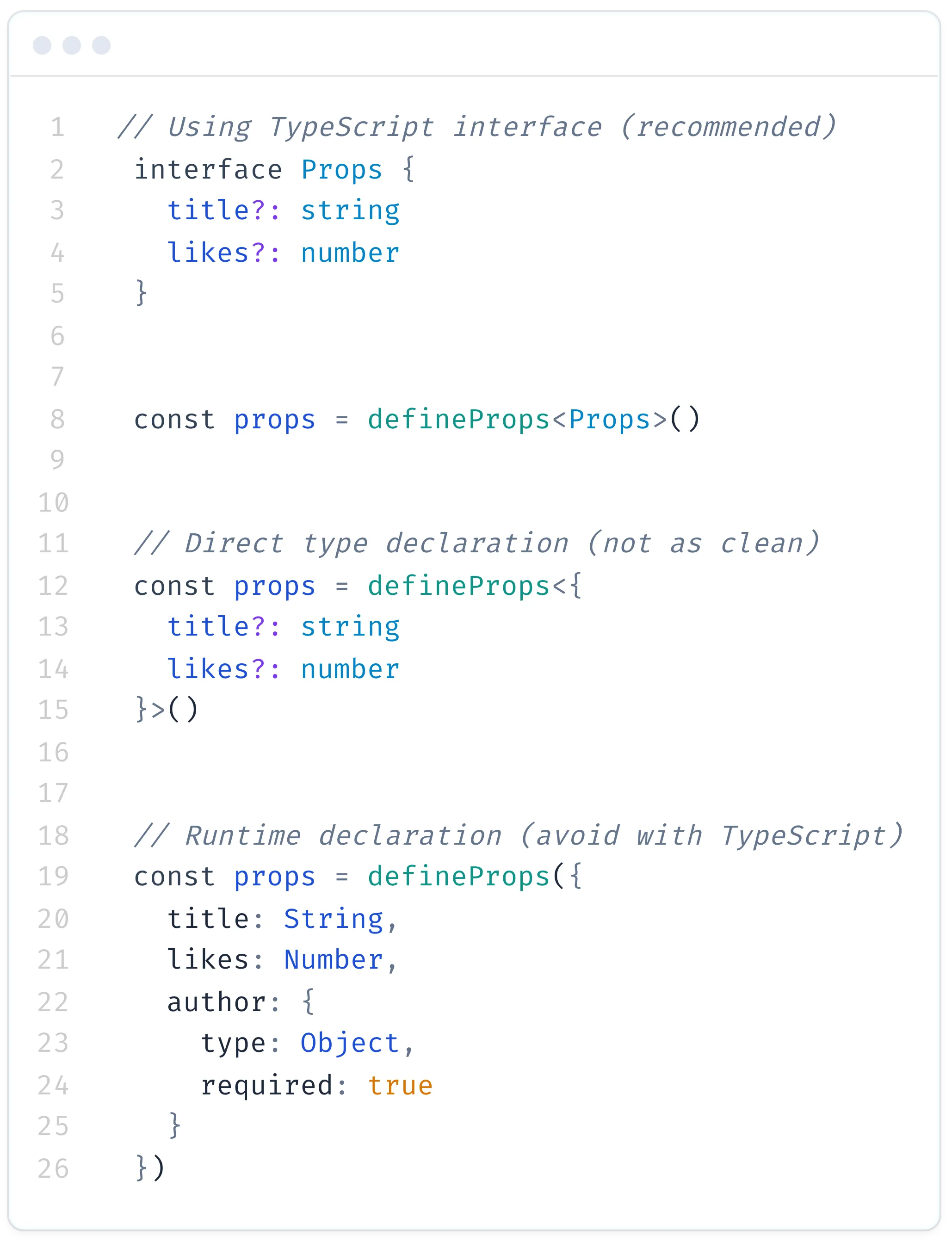
React
React takes a more JavaScript-centric approach:
- JSX feels natural for JavaScript developers
- Uses standard JavaScript expressions for conditionals and loops
- Props are passed like HTML attributes
- One main way to write components (function components with hooks)
Example of React syntax:
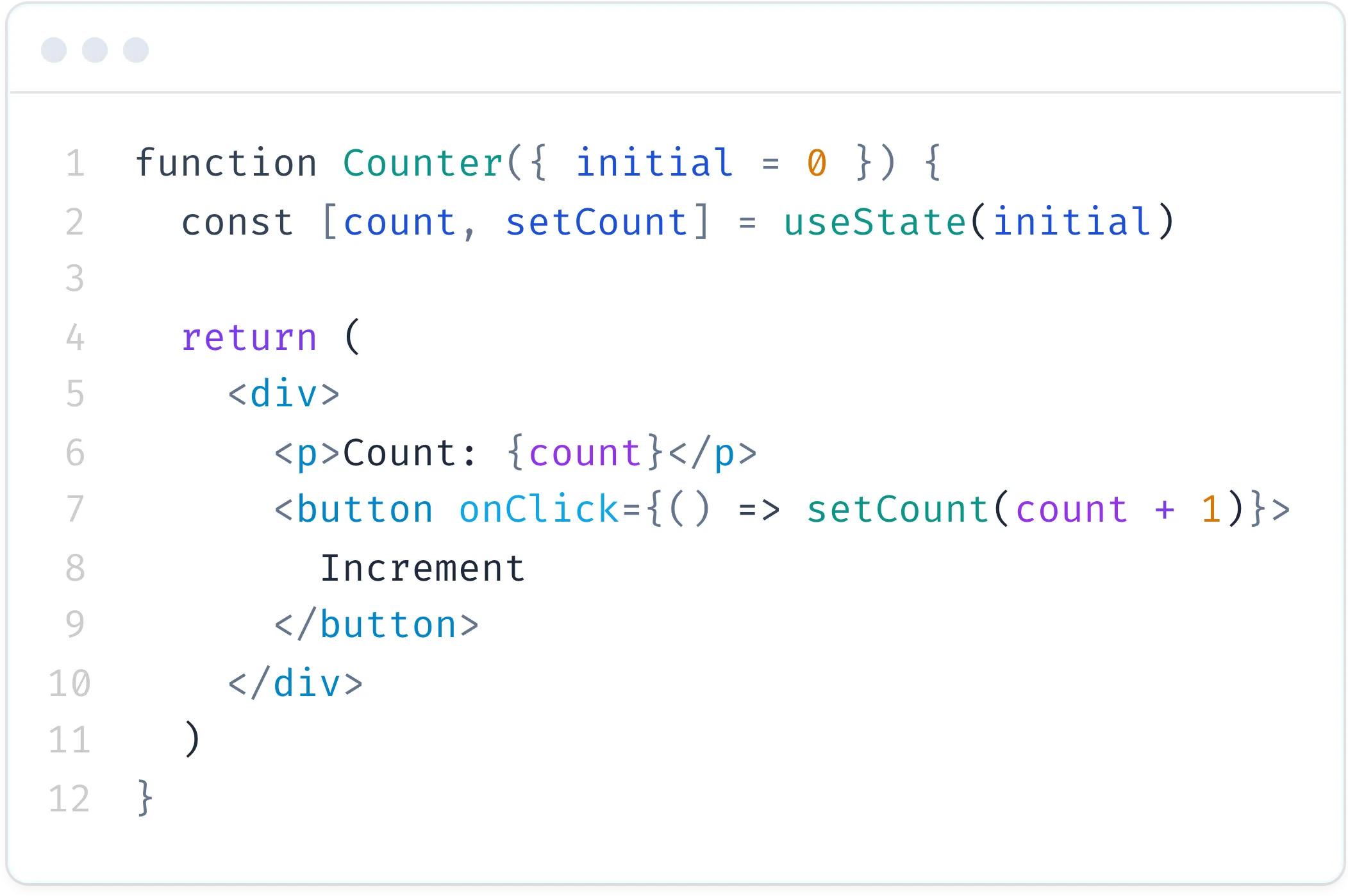
Disadvantages:
- Hooks can be confusing and error-prone
- useEffect dependencies and cleanup functions can be tricky and easy to forget
- Multiple hooks in the same component can be hard to reason about (it's often a code smell anyway)
- Conditional rendering and mapping components leads to verbose syntax
Outcome: As you can see, while Vue offers powerful syntactic Sugar, it has a learning curve and enforcing a consistent style across the codebase is not straightforward because of the variety of options. I personally recommend using the Composition API with TypeScript and defining the style rules that should be used across the codebase, such as how props should be defined or should :prop always be used instead of v-bind:prop. I think Vue has great ideas, but the syntax is not as clean as it could be.
React's syntax is more straightforward, if you already know Javascript and HTML. However, React has less syntactic sugar and the code can get more verbose, and harder to read. The hooks API can sometimes be confusing.
Vue's learning curve can be steeper due to its various shortcuts and APIs, but its template syntax can be more readable for complex rendering logic. While Vue's feature set is impressive, the variety of ways to do things can lead to inconsistent codebases unless carefully managed with strict team standards.
Both have their pros and cons, and it really comes down to personal preference. In my experience, React's limited syntactic options make it easier to maintain and write consistent code, even though it means dealing with more verbose syntax and missing some convenient features.
3. Ecosystem and Tooling
Honestly, React dominates in this category. Both frameworks have rich ecosystems but React's offering is simply unmatched by any other framework.
React
- Vercel & Next.js: The developer experience provided by Vercel and Next.js is great. Server components, streaming, edge functions, image optimization - everything (usually) works out of the box. The integration between Next.js and Vercel's platform is seamless.
- Component Libraries: shadcn/ui has changed how we build UIs in React, offering high-quality, customizable components that you own. Material UI, HeadlessUI, Chakra UI, and many others also provide decent solutions for any design need.
- Developer Tools: React Developer Tools are more mature and stable compared to Vue's devtools. My personal experience with Vue devtools was that it often crashed.
- Community & Support: React's community is massive. Any problem you encounter has probably been solved and documented somewhere. Finding experienced React developers is also much easier.
Vue
- Official Libraries: Vue's official libraries like Pinia and Vue Router are well-maintained and work great.
- Component Libraries: While Vue has ports of popular libraries (like shadcn-vue), they might lag behind their React counterparts and don't always maintain feature parity.
- Nuxt: While Nuxt is a solid framework for building server-side rendered (SSR) applications, it doesn't quite match the innovation pace of Next.js. The gap in features and developer experience is somewhat noticeable.
- Growing Community: Vue's community is passionate but smaller. Finding solutions to complex problems can take longer, and cutting-edge features often come to React first.
Outcome: React wins this category. While Vue's ecosystem is perfectly capable of building modern applications, React's tooling and ecosystem are more advanced, more stable, and more innovative.
4. Reactivity and State Management
Vue
Vue's approach to reactivity and state management is one of its strongest features:
- Built-in reactivity system that automatically tracks dependencies and updates components
- Two-way data binding with v-model makes form handling easier
- computed properties and watchers provide powerful reactive capabilities out of the box
- Pinia, Vue's state management solution, is awesome. It offers:
- Simple and intuitive API
- TypeScript support that works well
- Good balance between complexity and features
- Modular store design that scales well
Example of Pinia's syntax:
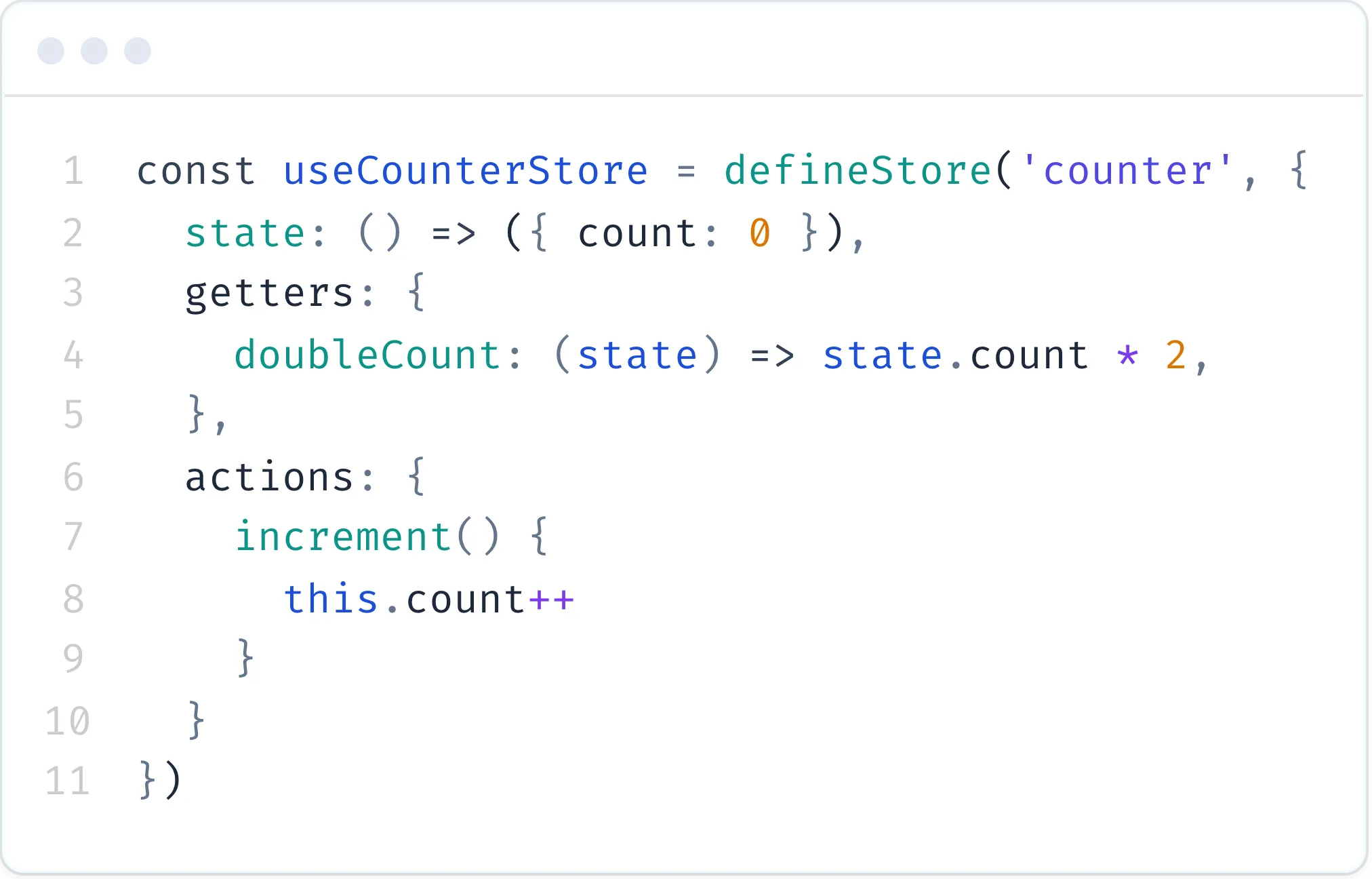
React
- React's state management landscape is more fragmented and often more complex:
- One-way data flow with props can lead to prop drilling issues
- No built-in way to handle computed values (need custom hooks or libraries)
- More boilerplate compared to Vue for patterns like two-way binding
- Context API is built-in but is not enough for complex state
- Multiple third-party solutions with different trade-offs:
- Redux Toolkit: Powerful but verbose, steepish learning curve
- Zustand: Simpler than Redux but type inference is not as good
- MobX: Good reactivity but feels out of place in React, and not as popular
- Jotai/Recoil: Atomic approach that can become complex
The lack of a standard solution in React's state management ecosystem often leads to decision paralysis and maintenance challenges.
Outcome: Vue is the clear winner here. Its reactive system is elegant and intuitive, and Pinia provides a state management solution that hits the sweet spot between simplicity and power. React's offerings either feel too complex (Redux), too simple (Context), or not quite right (other solutions). The fragmentation in React's state management ecosystem makes it harder to choose and standardize across projects. In simple CRUD apps, solutions such as Tanstack Query or SWR for managing server state are often better alternatives to heavier state management solutions.
5. Developer availability and experience
When it comes to developer availability, especially in Finland, React dominates the market. A quick job search in LinkedIn shows approximately 500 React positions compared to around 50 Vue positions in the Finnish market. This of course means there is more competition too. Differentiating by being a Vue company might be a good strategy to attract talented Vue developers.
The good news is that the learning curve between these frameworks isn't steep for experienced developers. A proficient React developer can become productive with Vue within 1-2 weeks, as the core concepts (components, props, state management) remain similar. The main adjustments involve:
- Learning Vue's template syntax and directives
- Understanding the Composition API (which is actually quite similar to React hooks)
- Getting familiar with Vue's reactivity system and Pinia state management
Outcome: React has a clear advantage in terms of developer availability in Finland. While Vue developers exist, the React talent pool is significantly larger. However, the relatively easy transition between frameworks means that hiring React developers and training them in Vue is a viable strategy if Vue better suits your project needs.
6. Common Ground - Shared Tooling
Several tools work equally well with both frameworks:
Testing
- Vitest: Modern testing framework
- Cypress: End-to-end testing framework
- Playwright: Microsoft's E2E testing tool
Build Tools
- Vite: Originally created for Vue but now excellent for React too
- Webpack: Still widely used with both frameworks despite newer alternatives
Developer Tools
- VS Code / Cursor: Excellent support for both with extensions
- Webstorm: Excellent support for both
- ESLint: Strong linting capabilities for both frameworks
- Prettier: Code formatting works equally well
- TypeScript: Both frameworks have good TypeScript support
Deployment & Hosting
- Vercel: While known for Next.js, works with Vue too
- Netlify: Support for both frameworks
- Railway: Works great with both frameworks
Development Utilities
- TanStack Query (formerly React Query): Available for both frameworks
- Storybook: Component documentation and testing for both
- Tailwind CSS: Works perfectly with both frameworks
- Sass/Less: CSS preprocessors work identically
This shared tooling means teams can often keep their testing, build, and deployment strategies consistent even when switching between frameworks. It also means that learning these tools is valuable regardless of which framework you choose.
Conclusion
While both Vue and React are capable frameworks, the choice between them generally comes down to specific team and project requirements rather than technical merits alone.
Choose Vue when:
- Your team (particularly tech leads) has strong Vue expertise
- You prefer building custom components and design systems rather than relying on third-party libraries
- Server-side rendering or server actions aren't critical requirements for your project
- You're building a Single Page Application (SPA) that needs to handle complex state management elegantly
- You vastly prefer Vue's reactivity system and syntactic magic over React's
Choose React when:
- You're building a new team or working with consultants (skilled React developers are more abundant)
- Your project relies heavily on third-party components, form and feature libraries
- You need advanced server-side rendering capabilities, server components, or want to leverage the Vercel/Next.js ecosystem
- You value flexibility and want access to the largest ecosystem of tools and libraries in the frontend world
- You need to hire developers quickly or want to ensure long-term maintainability with easily available talent
If you don't have strong preferences either way, React's larger ecosystem and developer availability often make it the better choice, especially in markets like Finland. Even though I enjoyed Vue's reactivity system and some of the syntactic sugar, React will also be my personal choice going forward, as I prefer its tooling and feel like it's a better long term bet. Companies such as Vercel and Meta are also investing heavily in React, and it shows no sign of slowing down or losing popularity.